How to use Dark Mode in Bootstrap
Use built-in color modes to toggle dark appearance in your website.
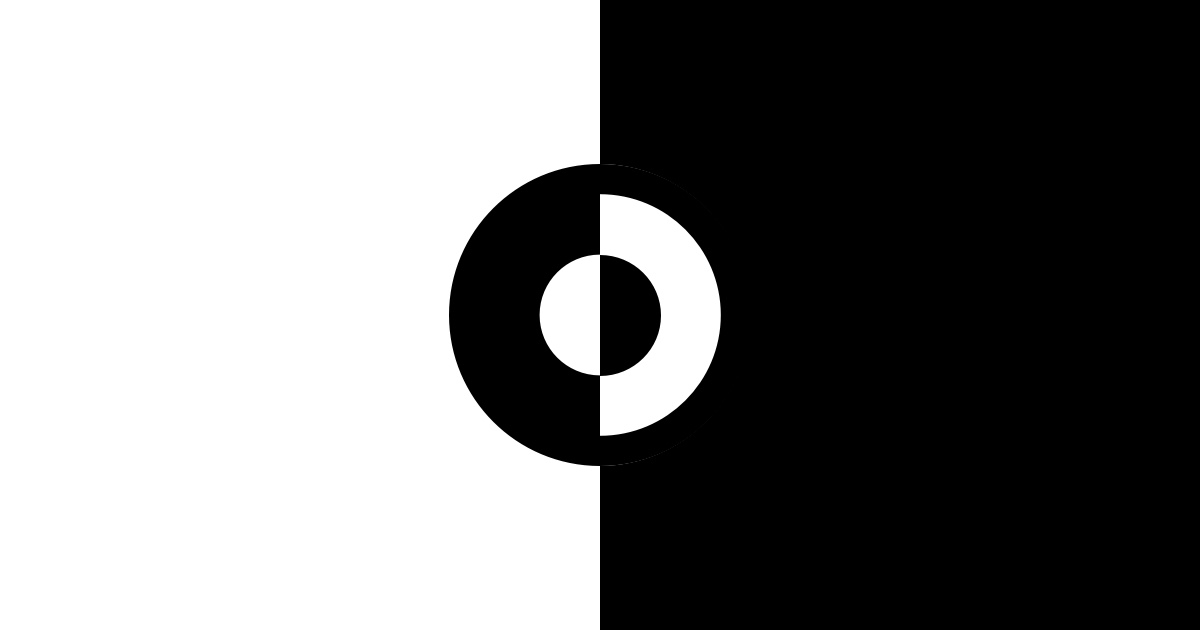
Introduction
Thanks to the new prefers-color-scheme
media query, automatic dark mode in websites has never been easier. But for developers using Bootstrap it wasn’t the case — until now. As of Bootstrap version 5.3.0, color modes are now supported, which means built-in support for dark mode! In this article, I will walk you through setting dark mode up and even toggling it automatically using JavaScript.
Prerequisites
To use color modes, you need to upgrade Bootstrap to v5.3 or above.
Include via NPM:
$ npm install bootstrap@5.3.0-alpha1
Alternatively, you can include the scripts by CDN:
https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css
https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js
Color Modes
Color modes (available in v5.3 and above) are basically a set of nice colors defined to match a theme. Light and dark modes are available out of the box, and you can create custom ones just by picking a set of color variables.
You can apply a color mode by adding a data-bs-theme
attribute:
<div data-bs-theme="dark" class="bg-body p-3">
<h1>This heading will appear in a dark theme.</h1>
<a href="#">This is a cool link in dark mode.</a>
</div>
<div class="bg-body p-3">
<h1>This heading will not be dark.</h1>
<a href="#">This link is sad because it is in light :(</a>
</div>
Global Themes
Color modes can also be applied to the whole page. To do that, add the theme attribute to the html
element. Note that nested elements with a different theme will override the global value. In the example below, the light theme of the h2
element overrides the dark theme of the html
element.
<html data-bs-theme="dark">
<head>{...}</head>
<body class="p-3">
<h1>This entire page is dark.</h1>
<p>This text is dark, too.</p>
<h2 data-bs-theme="light" class="bg-body text-body">
But this heading is *not* dark.
</h2>
</body>
</html>
Switching Modes
Bootstrap themes doesn’t have a built-in way to switch color modes, but with a little bit of JavaScript you can fully customize this behavior.
Automatically
This is what I did to this site. By adding an event listener to the media query, The theme will change instantly as the user sets the appearance.
// Bootstrap Dark Mode Switcher
// By Jongwoo Lee.
// Set theme to the user's preferred color scheme
function updateTheme() {
const colorMode = window.matchMedia("(prefers-color-scheme: dark)").matches
? "dark"
: "light";
document.querySelector("html").setAttribute("data-bs-theme", colorMode);
}
// Set theme on load
updateTheme()
// Update theme when the preferred scheme changes
window.matchMedia('(prefers-color-scheme: dark)').addEventListener('change', updateTheme)
By User Input
You may want to let the user pick their preferred theme, using a dropdown or toggle. This is also pretty much straightforward, though you will probably want to store the value in localStorage
to use it later.
Choose your theme:
<select id="themePicker">
<option value="light" selected>Light</option>
<option value="dark">Dark</option>
</select>
// Bootstrap Dark Mode Switcher
// By Jongwoo Lee.
let picker = document.getElementById("themePicker")
// Set theme to the user's preferred color scheme
function setTheme(newValue) {
document.querySelector("html").setAttribute("data-bs-theme", newValue);
}
// Set theme on load
setTheme(window.matchMedia("(prefers-color-scheme: dark)").matches
? "dark"
: "light")
// Update theme when the user selects something
picker.addEventListener('change', function() {
setTheme(picker.value)
})
Conclusion
That was all about enabling dark mode in Bootstrap, plus updating themes with JavaScript. Being one of the most popular frontend frameworks in the industry, this new feature would benefit a lot of users in dark mode. If you have any questions, comments or corrections, please feel free to email me at jongwoo@jongwoo.dev.